Analysis module¶
Collection of useful analysis tools. For easy acess use the Analysis facade
Analysis facade¶
Easy access to complex algorithmic concepts.
Author: | Sebastian Reinhard |
---|---|
Organization: | Biophysics and Biotechnology, Julius-Maximillians-University of Würzburg |
Version: | 2019.06.26 |
-
impro.analysis.analysis_facade.
create_alpha_shape
(storm_data: numpy.ndarray, alpha_value: float, px_size=32.2, line_width=1.0) → numpy.ndarray¶ Facade function to render alpha shape of dSTORM data
Image is rendered from (0,0) to the maximal dimension of the dSTORM data.
Parameters: - storm_data (np.ndarray(nx5)) – Point Cloud data to be rendered
- px_size (float) – Pixel size for the rendered image in nanometer per pixel
- alpha_value (float) – Core value to compute the alpha shape in nanometer
- line_width (float) – Line width of the alpha shape lines in a.u.
Returns: image – Rendered image
Return type: np.array
Example
>>> points = np.random.randint(0,32000,(1000000, 2)) >>> image = create_alpha_shape(points, 130) >>> image.shape (994,994)
-
impro.analysis.analysis_facade.
create_storm
(storm_data: numpy.ndarray, px_size=32.2, size=20, cluster=array(3.))¶ Facade function to render an image of dSTORM data.
Image is rendered from (0,0) to the maximal dimension of the dSTORM data.
Parameters: - storm_data (np.array(nx5)) – Point Cloud data to be rendered
- px_size (float) – Pixel size for the rendered image in nanometer per pixel
- size (float) – Size of the rendered points in nanometer
- cluster (np.array(n)) – Affiliation of the i th point to cluster[i] cluster
Returns: image – Rendered image
Return type: np.array
Example
>>> points = np.random.randint(0,32000,(1000000, 5)) >>> image = create_alpha_shape(points, 130) >>> image.shape (994,994)
-
impro.analysis.analysis_facade.
error_management
(result_list: list, source_points, target_points, n_row=5)¶ Test the results of th weighted GHT for missmatches
Parameters: - result_list (list) – results of the Weighted General Hough Transform
- source_points (np.array) – Source points of affine transformation
- target_points (np.array) – Target points of affine transformation
- n_row (int) – number of rows used for the weighted GHT
Returns: source_points, target_points – Filtered source and target points for affine transformation
Return type: np.array
-
impro.analysis.analysis_facade.
find_mapping
(target: numpy.ndarray, source_color: numpy.ndarray, n_col=5, n_row=5, offset=0) → numpy.ndarray¶ Facade function to find a mapping from source_color image to gray scale target image.
Parameters: - target (np.array) – Target image of affine transformation
- source_color (np.array) – Source image of affine transformation
- n_col (int) – number of collums to seperate the source image into
- n_row (int) – number of rows to seperate the source image into
- offset (int) – Start seperating the source image at (0+offset,0+offset). Offset is given in pixel
Returns: - source_points, target_points (np.array) – Source and target points for affine transformation
- overlay (np.array) – Target image overlayed with source image segments at the matching position
- results (list) – Results of the Weighted General Hough Transform
Example
>>> import cv2 >>> points = np.random.randint(0,32000,(1000000, 5)) >>> source = create_alpha_shape(points, 130) >>> points = np.random.randint(0,120000,(1000000, 5)) >>> target = create_alpha_shape(points, 130) >>> find_mapping(cv2.cvtColor(target, cv2.COLOR_RGBA2GRAY), source)
-
impro.analysis.analysis_facade.
pearson_correlation
(target: numpy.ndarray, source: numpy.ndarray, map: numpy.ndarray) → float¶ Compute pearson correlation index after alignment
Parameters: - target (np.array) – target image in gray scale
- source (np.array) – source image in gray scale
- map (sklearn.transformation) – computed transformation
Returns: source_points, target_points – Filtered source and target points for affine transformation
Return type: np.array
Example
>>> import skimage.transform >>> source = np.ones((1000,1000)) >>> target = np.ones((1000,1000)) >>> source_points = np.array([[1.0,1.0],[500,500],[700,500]) >>> target_points = source_points >>> M = transform.estimate_transform("affine",source_points,target_points) >>> pearson_correlation(target, source, M) (1.0)
Alpha Shape¶
The objective of the alpha shape algorithm is to deliver a formal meaning for the geometric notation of ‘shape’,
in the context of finite point sets.
The straciatella ice cream example gives a visual explanation of the concept:
Consider a ball of ice cream with chocolate pieces.
The chocolate pieces represent the distribution of points in space (ice cream).
The alpha shape algorithm now tries to eat as much ice cream as possible without touching any chocolate pieces,
using an arbitrary predefined spoon size, the -value. Choosing a very small spoon size results in all ice cream
being eaten, a very big spoon size in no ice cream being eaten at all (Convex Hull). But a size in between creates a
concave hull representing the shape of the distributed chocolate pieces, the desired alpha shape.
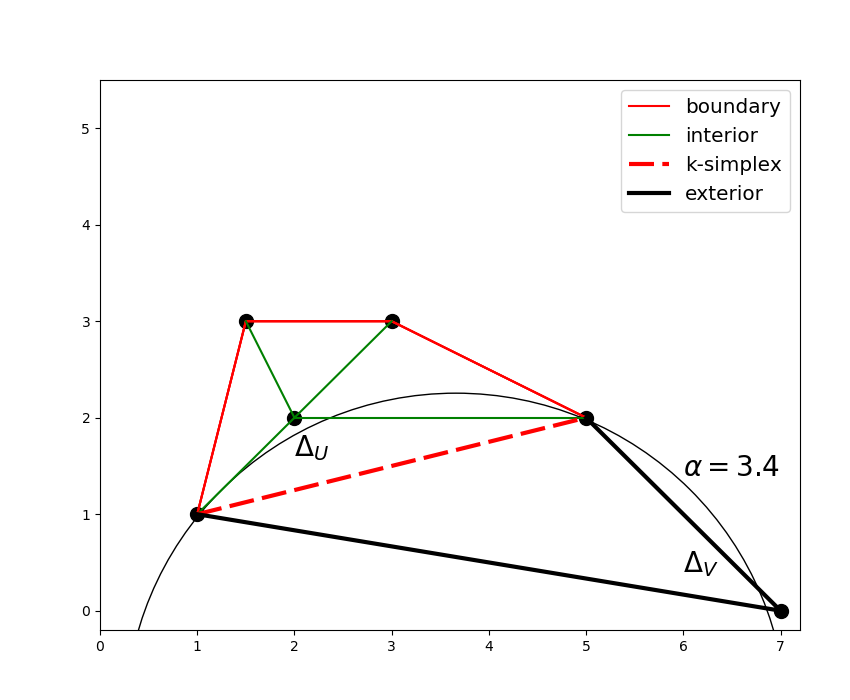
Considering the red dotted line l, the limit a is defined as the minimum of the diameter of the circumcircles around
and
. The limit b is the maximum of circumcircles around
and
.
Since the
-ball is smaller than b, but bigger than a, l is classified as boundary.
References
- Edelsbrunner, Herbert ; Mücke, Ernst P.: Three-dimensional Alpha Shapes. In: ACM Trans. Graph. 13 (1994), Januar, Nr. 1, 43–72. http: //dx.doi.org/10.1145/174462.156635. – DOI 10.1145/174462.156635. – ISSN 0730–0301
- Fischer, Kaspar: Introduction to Alpha Shapes. https: //graphics.stanford.edu/courses/cs268-11-spring/handouts/ AlphaShapes/as_fisher.pdf.
Author: | Sebastian Reinhard |
---|---|
Organization: | Biophysics and Biotechnology, Julius-Maximillians-University of Würzburg |
Version: | 2018.03.09 |
Example
>>> data = np.random.randint(0,32000,(1000000, 2))
>>> k_simplices = get_k_simplices(data[...,0:2])[0]
-
class
impro.analysis.alpha_shape_gpu.
AlphaComplex
(struct_ptr: int, indices: numpy.ndarray, points: numpy.ndarray, neighbors: numpy.ndarray)¶ Bases:
object
Derive 2D alpha complex from scipy.spatial Delaunay
Class to create a alpha complex structure on GPU.
Parameters: - struct_ptr (int) – pointer to allocated memmory for structure
- indices (np.array) – nx3 array containing point indices of the delaunay triangulation simplices
- neighbors (np.array) – nx3 array containing the indices of neighboring simplices
- points (np.array) – nx2 array of the points used for delaunay triangulation
-
get
()¶ Returns: nx5 of d=1 simplices containing: [index1, index2, dist, sigma1, sigma2] with sigma 1 < sigma 2 Return type: numpy.array
-
memsize
= 32¶
-
merge
()¶
-
impro.analysis.alpha_shape_gpu.
get_k_simplices
(points: numpy.ndarray)¶ Parameters: points (np.array) – nx2 array of points to use for alpha complex Returns: - alpha complex (mx5 array of d=1 simplices containing the upper and lower limits for a simplice to be interior/)
- on boundary of the alpha shape.
Filter¶
Author: | Sebastian Reinhard |
---|---|
Organization: | Biophysics and Biotechnology, Julius-Maximillians-University of Würzburg |
Version: | 2019.06.26 |
-
class
impro.analysis.filter.
Filter
¶ Bases:
object
-
static
local_density_filter
(points, r, n)¶ Get the indices of all points having a minimum of n neighbors in a radius of r
Parameters: - points (np.array) – Points to filter (X,Y,Z)
- r (float) – Search for neighbors in a radius of r
- n (int) – Required neighbors in radius r to pass the filter
Returns: filt – indices of entries passing the filter
Return type: np.array
-
static
values_filter
(value, minimum, maximum)¶ Get the indices of an array values with value > minimum and value < maximum
Parameters: - value (np.array) – Array with values to filter
- minimum (float) – minimum value to pass the filter
- maximum (float) – maximum value to pass the filter
Returns: filt – indices of entries passing the filter
Return type: np.array
-
static
Hough Transform¶
The General Hough Transform (GHT) maps the orientation of edge points in a template image to a predefined origin (typically the middle pixel of the image). Comparing this map with an image gives each point a rating of likelihood for being a source point of that map.
Author: | Sebastian Reinhard |
---|---|
Organization: | Biophysics and Biotechnology, Julius-Maximillians-University of Würzburg |
Version: | 2019.06.26 |
Example
>>> target = cv2.imread("path_to_file")
>>> template = cv2.imread("path_to_file")
>>> ght_target = GHTImage(target, blur=5, canny_lim=(130,180))
>>> H = HoughTransform()
>>> H.target = ght_target
>>> ght_template = TemplateImage(template)
>>> H.template = ght_template
>>> res = H.transform()
-
class
impro.analysis.hough_transform_gpu.
GHTImage
(image, blur=4, canny_lim=(130, 200))¶ Bases:
object
GHT specific image preprocessing
-
o_image
¶ Input image
Type: np.array
-
image
¶ Resized and blurred input image
Type: np.array
-
canny
¶ Canny Edge processed image
Type: np.array
-
gradient
¶ Gradient image
Type: np.array
-
-
class
impro.analysis.hough_transform_gpu.
HoughTransform
(rotation_min=-10, rotation_max=10)¶ Bases:
object
Perform Gradient Weighted General Hough Transform on the Graphics Processing Unit
-
rotation_min
¶ Start matching template at rotation_min
Type: float
-
rotation_max
¶ End matching template at rotation_max
Type: float
-
template
¶ Matching template image on target image
Type: np.array
-
target
¶ Matching template image on target image
Type: np.array
-
weighted
¶ Weight the GHT by Gradient density
Type: bool
-
transform
()¶ Perform GHT algorithm with given data
-
rotation_max
-
rotation_min
-
target
-
template
-
transform
()
-
-
class
impro.analysis.hough_transform_gpu.
Matrix
(array)¶ Bases:
object
Wrapper class for matrix and matrixf struct on GPU:
-
get
()¶
-
-
class
impro.analysis.hough_transform_gpu.
TemplateImage
(image, **kwargs)¶ Bases:
impro.analysis.hough_transform_gpu.GHTImage
Extend GHTImage by templte properties
-
o_image
¶ Input image
Type: np.array
-
image
¶ Resized and blurred input image
Type: np.array
-
canny
¶ Canny Edge processed image
Type: np.array
-
gradient
¶ Gradient image
Type: np.array
-
r_matrix_zero
¶ Vector mapping of edge points to a predefined origin
Type: np.array
-
r_matrix
¶ Rotated r_matrix_zero
Type: np.array
-
rotate_r_matrix
(angle)¶ Rotate R-Matrix by angle rad
- angle: float
- Angle to rotate matrix in rad
-